Boost Your Knowledge: JavaScript OOPs Interview Questions
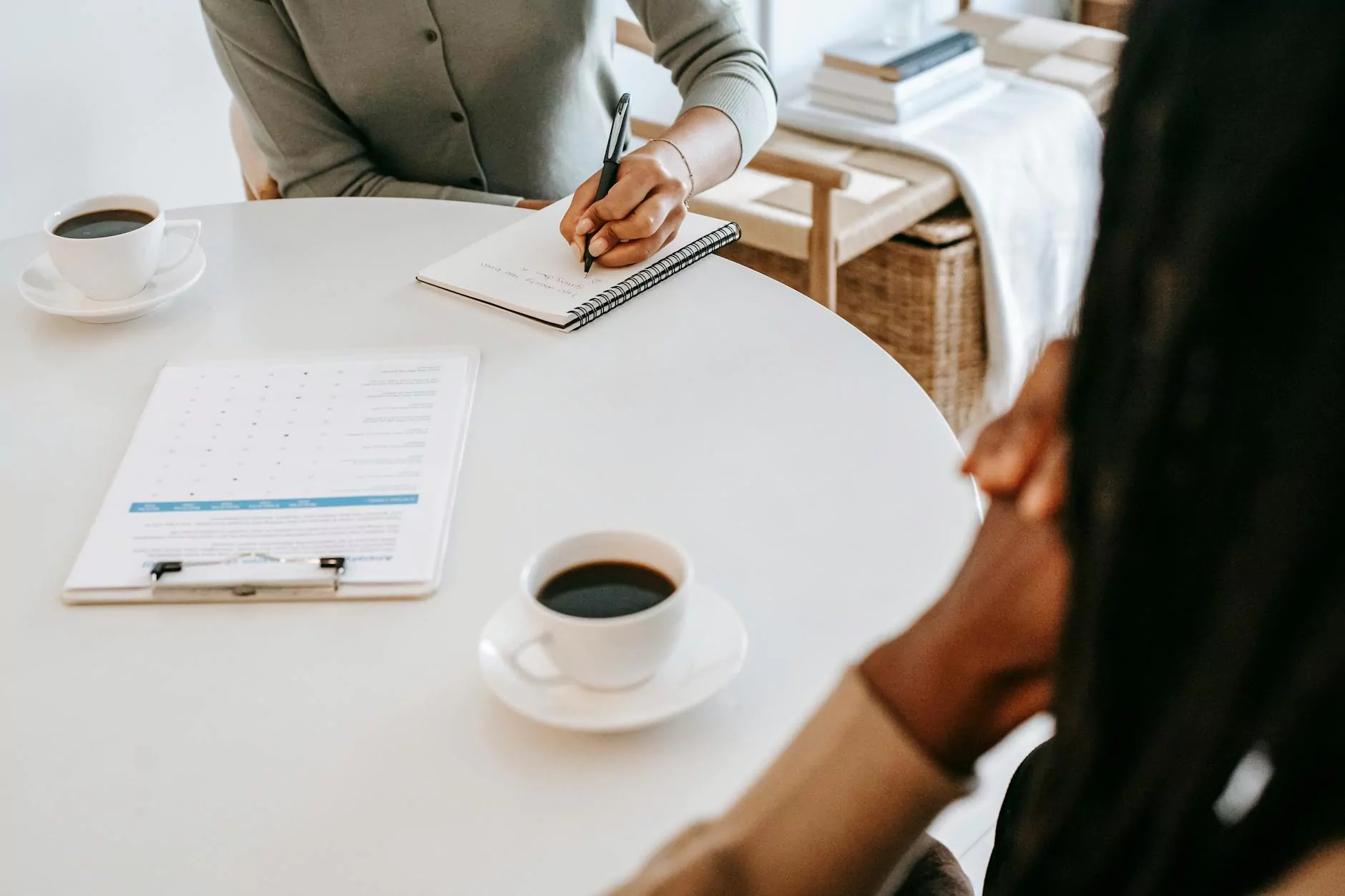
Welcome to Code-Sample.com, your go-to resource for all things related to IT services, computer repair, web design, and software development. In this article, we'll delve deep into JavaScript Object-Oriented Programming (OOPs) interview questions to help you excel in your next interview. Whether you're a beginner or an experienced developer, mastering JavaScript OOPs concepts is essential in today's competitive job market.
Understanding Object-Oriented Programming (OOP)
To grasp JavaScript OOPs interview questions, it's important to understand the fundamental principles of Object-Oriented Programming. OOP is a programming paradigm that organizes code into reusable objects. These objects are instances of a class, which serves as a blueprint defining their properties and behaviors.
In JavaScript, we can create objects using function constructors, the class keyword introduced in ES6, or object literals. Each approach has its own syntax and benefits, but the underlying OOP concepts remain the same.
Essential JavaScript OOPs Interview Questions
Here are some commonly asked JavaScript OOPs interview questions and detailed answers:
1. What is the difference between class-based and prototype-based inheritance?
Class-based inheritance involves creating objects based on pre-defined classes, where objects inherit properties and behaviors from their class. On the other hand, prototype-based inheritance allows objects to inherit directly from other objects, known as prototypes.
In JavaScript, we primarily use prototype-based inheritance. Objects have a hidden [[Prototype]] property, which links them to their prototypes, enabling inheritance.
2. How do you create an object in JavaScript?
In JavaScript, there are multiple ways to create objects:
- Using a function constructor: function Person(name) { this.name = name; this.greet = function() { console.log('Hello, ' + this.name); } }
- Using the class keyword: class Person { constructor(name) { this.name = name; } greet() { console.log('Hello, ' + this.name); } }
- Using object literals: const john = { name: 'John', greet: function() { console.log('Hello, ' + this.name); } };
To create an object:
const john = new Person('John');To create an object:
const john = new Person('John');3. Explain the concept of prototype in JavaScript.
In JavaScript, every object has a prototype. The prototype is an object from which the current object inherits properties and methods. When accessing a property or method on an object, JavaScript first checks if the object itself has it. If not, it looks up the prototype chain until it finds the property or method.
For example, consider the following code:
function Person(name) { this.name = name; } Person.prototype.greet = function() { console.log('Hello, ' + this.name); }; const john = new Person('John'); john.greet(); // Output: Hello, JohnIn this code snippet, Person.prototype is the prototype of the john object. The greet() method is defined on the prototype, allowing all instances of Person to access and invoke it.
4. What are the benefits of using OOP in JavaScript?
Object-Oriented Programming in JavaScript offers several advantages:
- Reusability: Objects and classes promote code reusability, allowing you to write cleaner and more maintainable code.
- Modularity: OOP enables you to encapsulate related properties and behaviors within objects, making your code modular and easier to reason about.
- Inheritance: JavaScript's prototype-based inheritance allows objects to inherit from other objects, fostering code reuse and preventing duplication.
- Abstraction: By defining classes and objects with specific interfaces, you can abstract away implementation details, allowing for better separation of concerns.
- Polymorphism: Polymorphism allows objects of different classes to share the same interface. This flexibility enables code to work with multiple object types interchangeably.
5. How does encapsulation relate to OOP?
Encapsulation is a core principle of OOP that involves bundling related properties and behaviors within objects. It allows you to control the access and visibility of object members, protecting data integrity and improving code maintainability.
In JavaScript, you can achieve encapsulation through the use of closures, module patterns, or the class syntax introduced in ES6. By defining private properties and methods, you limit external access, enabling objects to maintain their internal state.
Conclusion
Congratulations! You've delved into JavaScript OOPs interview questions, expanding your knowledge of Object-Oriented Programming in JavaScript. We covered essential concepts such as class-based vs. prototype-based inheritance, object creation, prototypes, and the benefits of using OOP.
Remember, the key to excelling in your next interview is not just memorizing answers but truly understanding the underlying principles. By practicing these concepts and exploring real-world scenarios, you'll be well-prepared to tackle any JavaScript OOPs interview question that comes your way!
Stay tuned for more informative articles and tutorials on Code-Sample.com, your trusted source for IT services, computer repair, web design, and software development.